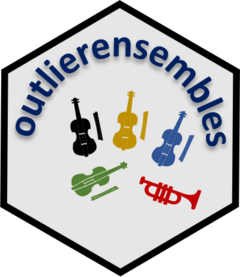
Computes an ensemble score using inverse cluster weighted averaging method by Chiang et al (2017)
icwa_ensemble.Rd
This function computes an ensemble score using inverse cluster weighted averaging in the paper titled A Study on Anomaly Detection Ensembles by Chiang et al (2017) <doi:10.1016/j.jal.2016.12.002>. The ensemble is detailed in Algorithm 2.
Examples
set.seed(123)
if (requireNamespace("dbscan", quietly = TRUE)) {
X <- data.frame(x1 = rnorm(200), x2 = rnorm(200))
X[199, ] <- c(4, 4)
X[200, ] <- c(-3, 5)
# Using different parameters of lof for anomaly detection
y1 <- dbscan::lof(X, minPts = 10)
y2 <- dbscan::lof(X, minPts = 20)
knnobj <- dbscan::kNN(X, k = 20)
# Using different KNN distances as anomaly scores
y3 <- knnobj$dist[ ,10]
y4 <- knnobj$dist[ ,20]
# Dense points are less anomalous. Hence 1 - pointdensity is used.
y5 <- 1 - dbscan::pointdensity(X, eps = 0.8, type = "gaussian")
y6 <- 1 - dbscan::pointdensity(X, eps = 0.5, type = "gaussian")
Y <- cbind.data.frame(y1, y2, y3, y4, y5, y6)
ens <- icwa_ensemble(Y)
ens
}
#> [1] 1.25267222 0.73960151 0.96579933 0.22902383 0.06307445 1.11084580
#> [7] 0.37601036 0.84430279 0.93681982 0.16640394 0.72685751 0.18146148
#> [13] 0.80555098 0.10459373 0.55237753 1.45730162 0.18758412 1.33733986
#> [19] 0.88384359 0.70513611 0.69884754 0.26752871 0.76456918 0.50586369
#> [25] 0.36368786 1.01026730 0.54555390 0.23194336 0.76916140 1.03867128
#> [31] 1.26176100 0.08599129 0.50159578 0.59971307 0.46187396 0.93535184
#> [37] 0.18465515 0.13802923 0.14270655 0.36016604 0.52277041 0.16515339
#> [43] 1.03465657 1.52238756 0.67482096 1.16975818 0.14626793 0.75353759
#> [49] 0.47522828 0.17439520 0.06974459 0.14408171 0.05496780 0.82435955
#> [55] 0.92672067 0.92607806 1.07407191 0.48364807 0.02447762 0.91904124
#> [61] 0.16603162 0.31113142 0.10020034 0.83038325 1.36278723 0.99859135
#> [67] 0.12689601 1.02887427 0.47689753 1.29028541 0.47105486 1.51103967
#> [73] 0.77158551 0.86905774 0.52587690 0.57547862 0.09164934 0.70200192
#> [79] 0.18951875 0.03284610 0.95585421 0.45290910 0.19272811 0.24284357
#> [85] 0.05576226 0.12904103 0.64857586 1.08818637 0.12494436 0.68163984
#> [91] 1.02925413 0.73918438 0.69855788 0.40666633 1.45676000 0.28408932
#> [97] 1.59435220 1.32635089 0.05328998 0.81257910 0.49838093 0.28089142
#> [103] 0.41823496 0.51575461 0.58045516 0.09873506 1.28103807 1.00167474
#> [109] 0.67895132 1.40274076 0.72277688 0.56543396 1.49404302 0.16508954
#> [115] 0.17315957 0.39609860 0.02348507 0.75833685 0.97821468 0.71124036
#> [121] 0.08605348 0.76991364 0.74266484 0.30219008 1.20467770 0.46475313
#> [127] 0.04853310 0.28706466 0.82596816 0.02542926 1.17752349 0.73324164
#> [133] 0.22745576 0.86058373 1.28327317 1.46046149 1.27709514 0.31379549
#> [139] 1.34033356 0.85099739 0.54884964 0.50099936 1.26568142 0.88971131
#> [145] 0.95808409 1.03821518 0.85105428 0.35940315 1.44916462 0.75964219
#> [151] 0.82490029 1.34051083 0.11146059 0.58174199 0.37037186 0.13593018
#> [157] 0.35992200 0.11665458 1.59641104 1.45225666 0.55474218 0.66556800
#> [163] 0.74249437 1.79234934 0.43138899 0.05943459 0.39245898 0.49429633
#> [169] 0.59417992 0.13795534 1.38173520 0.95647803 0.09721340 1.41901632
#> [175] 0.45671515 0.72405942 0.45434508 0.12405036 0.13882992 1.04445811
#> [181] 0.60770027 0.77551530 0.11756709 0.74782551 0.18931022 0.78741108
#> [187] 0.73967357 0.57966077 0.34864899 0.25358447 0.18943031 1.28257083
#> [193] 0.47148648 0.66175629 0.86893218 1.47242890 0.43934565 0.83172210
#> [199] 2.41525124 2.65965710