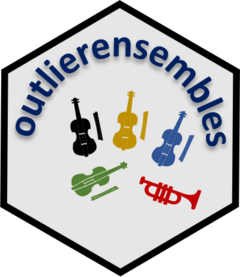
Computes an ensemble score using the maximum score of each observation
max_ensemble.Rd
This function computes an ensemble score using the maximum score for each observation as detailed in Aggarwal and Sathe (2015) <doi:10.1145/2830544.2830549>.
Examples
set.seed(123)
if (requireNamespace("dbscan", quietly = TRUE)) {
X <- data.frame(x1 = rnorm(200), x2 = rnorm(200))
X[199, ] <- c(4, 4)
X[200, ] <- c(-3, 5)
# Using different parameters of lof for anomaly detection
y1 <- dbscan::lof(X, minPts = 10)
y2 <- dbscan::lof(X, minPts = 20)
knnobj <- dbscan::kNN(X, k = 20)
# Using different KNN distances as anomaly scores
y3 <- knnobj$dist[ ,10]
y4 <- knnobj$dist[ ,20]
# Dense points are less anomalous. Hence 1 - pointdensity is used.
y5 <- 1 - dbscan::pointdensity(X, eps = 0.8, type = "gaussian")
y6 <- 1 - dbscan::pointdensity(X, eps = 0.5, type = "gaussian")
Y <- cbind.data.frame(y1, y2, y3, y4, y5, y6)
ens <- max_ensemble(Y)
ens
}
#> [1] 0.85597815 0.56501055 0.70579251 0.18802914 0.04197203 0.78779628
#> [7] 0.28925776 0.64296176 0.68692157 0.14674795 0.55563568 0.13756610
#> [13] 0.62192229 0.07711241 0.43418522 0.92107929 0.14053063 0.91073722
#> [19] 0.64960328 0.53752328 0.54889545 0.22241477 0.57943870 0.39590696
#> [25] 0.28260492 0.73375426 0.42064082 0.17959946 0.58699920 0.75001744
#> [31] 0.87007019 0.07602438 0.39749784 0.45897682 0.35572621 0.68619296
#> [37] 0.13574944 0.11943692 0.12752965 0.28996935 0.41901683 0.12371377
#> [43] 0.73991947 0.94044897 0.52104285 0.81474544 0.12686339 0.57020375
#> [49] 0.36869446 0.14977279 0.04826159 0.10584581 0.03452781 0.62493435
#> [55] 0.68808191 0.68244157 0.77721325 0.39476023 0.02578896 0.67593447
#> [61] 0.12420196 0.24388157 0.09118399 0.61920779 0.90265631 0.73470263
#> [67] 0.08904537 0.74625313 0.36684299 0.88755168 0.36831545 0.95910811
#> [73] 0.60357782 0.64470140 0.41815623 0.44359055 0.08686416 0.55226325
#> [79] 0.15407786 0.02632366 0.70497668 0.37021905 0.16741410 0.18496622
#> [85] 0.05620216 0.09176927 0.50814584 0.78600033 0.10200134 0.52384349
#> [91] 0.75684927 0.57907961 0.54682222 0.32937334 0.93654296 0.23344276
#> [97] 0.95352985 0.89846128 0.05222640 0.60928269 0.40573926 0.21340175
#> [103] 0.32913648 0.40389850 0.46724921 0.08464179 0.86661530 0.73175606
#> [109] 0.52286633 0.92094304 0.55138822 0.46168503 0.94981017 0.12758279
#> [115] 0.13021472 0.32598315 0.02736458 0.56952419 0.70920464 0.54619953
#> [121] 0.06533857 0.57956791 0.56535826 0.24737731 0.83251688 0.36091435
#> [127] 0.02508661 0.23591866 0.61500780 0.02076423 0.83334821 0.54567025
#> [133] 0.17903888 0.64098202 0.87405419 0.93521553 0.87826667 0.23753250
#> [139] 0.89562808 0.64350669 0.44258525 0.39568804 0.86544905 0.66707184
#> [145] 0.70309446 0.74236809 0.64762234 0.27856622 0.91992371 0.58733561
#> [151] 0.63857198 0.89806629 0.07822828 0.46013064 0.28726347 0.12254420
#> [157] 0.29258158 0.10846899 0.96134686 0.92872752 0.42859421 0.52286600
#> [163] 0.57846259 0.99633536 0.34282165 0.03340332 0.31508673 0.39070302
#> [169] 0.47545946 0.09927660 0.91148361 0.70548502 0.06827707 0.92438241
#> [175] 0.36155551 0.56530297 0.34563040 0.08762209 0.09872697 0.74837911
#> [181] 0.48392377 0.58867958 0.11237191 0.58073566 0.16184674 0.59867684
#> [187] 0.57857451 0.43683133 0.27198736 0.21120867 0.14682390 0.86908051
#> [193] 0.37872663 0.52683127 0.65885653 0.92419194 0.33679684 0.63003414
#> [199] 1.00000000 1.00000000